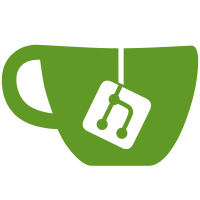
index id's are now opaque (until finally returned to top-level user) - the TermFieldDoc's returned by TermFieldReader no longer contain doc id - instead they return an opaque IndexInternalID - items returned are still in the "natural index order" - but that is no longer guaranteed to be "doc id order" - correct behavior requires that they all follow the same order - but not any particular order - new API FinalizeDocID which converts index internal ID's to public string ID - APIs used internally which previously took doc id now take IndexInternalID - that is DocumentFieldTerms() and DocumentFieldTermsForFields() - however, APIs that are used externally do not reflect this change - that is Document() - DocumentIDReader follows the same changes, but this is less obvious - behavior clarified, used to iterate doc ids, BUT NOT in doc id order - method STILL available to iterate doc ids in range - but again, you won't get them in any meaningful order - new method to iterate actual doc ids from list of possible ids - this was introduced to make the DocIDSearcher continue working searchers now work with the new opaque index internal doc ids - they return new DocumentMatchInternal (which does not have string ID) scorerers also work with these opaque index internal doc ids - they return DocumentMatchInternal (which does not have string ID) collectors now also perform a final step of converting the final result - they STILL return traditional DocumentMatch (with string ID) - but they now also require an IndexReader (so that they can do the conversion)
60 lines
1.7 KiB
Go
60 lines
1.7 KiB
Go
// Copyright (c) 2014 Couchbase, Inc.
|
|
// Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
|
|
// except in compliance with the License. You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
// Unless required by applicable law or agreed to in writing, software distributed under the
|
|
// License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
|
|
// either express or implied. See the License for the specific language governing permissions
|
|
// and limitations under the License.
|
|
|
|
package scorers
|
|
|
|
import (
|
|
"github.com/blevesearch/bleve/search"
|
|
)
|
|
|
|
type ConjunctionQueryScorer struct {
|
|
explain bool
|
|
}
|
|
|
|
func NewConjunctionQueryScorer(explain bool) *ConjunctionQueryScorer {
|
|
return &ConjunctionQueryScorer{
|
|
explain: explain,
|
|
}
|
|
}
|
|
|
|
func (s *ConjunctionQueryScorer) Score(constituents []*search.DocumentMatchInternal) *search.DocumentMatchInternal {
|
|
rv := search.DocumentMatchInternal{
|
|
ID: constituents[0].ID,
|
|
}
|
|
|
|
var sum float64
|
|
var childrenExplanations []*search.Explanation
|
|
if s.explain {
|
|
childrenExplanations = make([]*search.Explanation, len(constituents))
|
|
}
|
|
|
|
locations := []search.FieldTermLocationMap{}
|
|
for i, docMatch := range constituents {
|
|
sum += docMatch.Score
|
|
if s.explain {
|
|
childrenExplanations[i] = docMatch.Expl
|
|
}
|
|
if docMatch.Locations != nil {
|
|
locations = append(locations, docMatch.Locations)
|
|
}
|
|
}
|
|
rv.Score = sum
|
|
if s.explain {
|
|
rv.Expl = &search.Explanation{Value: sum, Message: "sum of:", Children: childrenExplanations}
|
|
}
|
|
|
|
if len(locations) == 1 {
|
|
rv.Locations = locations[0]
|
|
} else if len(locations) > 1 {
|
|
rv.Locations = search.MergeLocations(locations)
|
|
}
|
|
|
|
return &rv
|
|
}
|