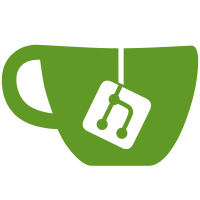
In the index/store package introduce KVReader creates snapshot all read operations consistent from this snapshot must close to release introduce KVWriter only one writer active access to all operations allows for consisten read-modify-write must close to release introduce AssociativeMerge operation on batch allows efficient read-modify-write for associative operations used to consolidate updates to the term summary rows saves 1 set and 1 get op per shared instance of term in field In the index package introduced an IndexReader exposes a consisten snapshot of the index for searching At top level All searches now operate on a consisten snapshot of the index
54 lines
1.5 KiB
Go
54 lines
1.5 KiB
Go
// Copyright (c) 2014 Couchbase, Inc.
|
|
// Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
|
|
// except in compliance with the License. You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
// Unless required by applicable law or agreed to in writing, software distributed under the
|
|
// License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
|
|
// either express or implied. See the License for the specific language governing permissions
|
|
// and limitations under the License.
|
|
|
|
package bleve
|
|
|
|
import (
|
|
"github.com/blevesearch/bleve/index"
|
|
"github.com/blevesearch/bleve/search"
|
|
"github.com/blevesearch/bleve/search/searchers"
|
|
)
|
|
|
|
type matchAllQuery struct {
|
|
BoostVal float64 `json:"boost,omitempty"`
|
|
}
|
|
|
|
// NewMatchAllQuery creates a Query which will
|
|
// match all documents in the index.
|
|
func NewMatchAllQuery() *matchAllQuery {
|
|
return &matchAllQuery{
|
|
BoostVal: 1.0,
|
|
}
|
|
}
|
|
|
|
func (q *matchAllQuery) Boost() float64 {
|
|
return q.BoostVal
|
|
}
|
|
|
|
func (q *matchAllQuery) SetBoost(b float64) Query {
|
|
q.BoostVal = b
|
|
return q
|
|
}
|
|
|
|
func (q *matchAllQuery) Searcher(i index.IndexReader, m *IndexMapping, explain bool) (search.Searcher, error) {
|
|
return searchers.NewMatchAllSearcher(i, q.BoostVal, explain)
|
|
}
|
|
|
|
func (q *matchAllQuery) Validate() error {
|
|
return nil
|
|
}
|
|
|
|
func (q *matchAllQuery) Field() string {
|
|
return ""
|
|
}
|
|
|
|
func (q *matchAllQuery) SetField(f string) Query {
|
|
return q
|
|
}
|