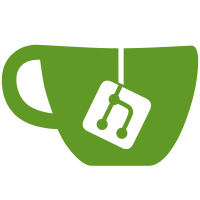
this improves perf in the case where we're not doing any sorting as we avoid allocating memory and converting scores into numeric terms
38 lines
835 B
Go
38 lines
835 B
Go
package collectors
|
|
|
|
import (
|
|
"math/rand"
|
|
"strconv"
|
|
"testing"
|
|
|
|
"github.com/blevesearch/bleve/index"
|
|
"github.com/blevesearch/bleve/search"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
type createCollector func() search.Collector
|
|
|
|
func benchHelper(numOfMatches int, cc createCollector, b *testing.B) {
|
|
dp := search.NewDocumentMatchPool(numOfMatches, 1)
|
|
matches := make([]*search.DocumentMatch, 0, numOfMatches)
|
|
for i := 0; i < numOfMatches; i++ {
|
|
match := dp.Get()
|
|
match.IndexInternalID = index.IndexInternalID(strconv.Itoa(i))
|
|
match.Score = rand.Float64()
|
|
matches = append(matches, match)
|
|
}
|
|
|
|
b.ResetTimer()
|
|
|
|
for run := 0; run < b.N; run++ {
|
|
searcher := &stubSearcher{
|
|
matches: matches,
|
|
}
|
|
collector := cc()
|
|
err := collector.Collect(context.Background(), searcher, &stubReader{})
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|