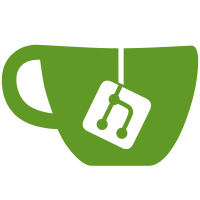
as we are a Go library is this the much more natural way to express such queries. support for strings is still supported through json marshal and unmarshal, as well as inside query string queries as before we use the package level QueryDateTimeParser to deterimine which date time parser to use for parsing only serializing out to json, we consult a new package variable: QueryDateTimeFormat this addresses the longstanding PR #255
198 lines
7.3 KiB
Go
198 lines
7.3 KiB
Go
// Copyright (c) 2014 Couchbase, Inc.
|
|
// Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
|
|
// except in compliance with the License. You may obtain a copy of the License at
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
// Unless required by applicable law or agreed to in writing, software distributed under the
|
|
// License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
|
|
// either express or implied. See the License for the specific language governing permissions
|
|
// and limitations under the License.
|
|
|
|
package bleve
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/blevesearch/bleve/search/query"
|
|
)
|
|
|
|
// NewBoolFieldQuery creates a new Query for boolean fields
|
|
func NewBoolFieldQuery(val bool) *query.BoolFieldQuery {
|
|
return query.NewBoolFieldQuery(val)
|
|
}
|
|
|
|
// NewBooleanQuery creates a compound Query composed
|
|
// of several other Query objects.
|
|
// These other query objects are added using the
|
|
// AddMust() AddShould() and AddMustNot() methods.
|
|
// Result documents must satisfy ALL of the
|
|
// must Queries.
|
|
// Result documents must satisfy NONE of the must not
|
|
// Queries.
|
|
// Result documents that ALSO satisfy any of the should
|
|
// Queries will score higher.
|
|
func NewBooleanQuery() *query.BooleanQuery {
|
|
return query.NewBooleanQuery(nil, nil, nil)
|
|
}
|
|
|
|
// NewConjunctionQuery creates a new compound Query.
|
|
// Result documents must satisfy all of the queries.
|
|
func NewConjunctionQuery(conjuncts ...query.Query) *query.ConjunctionQuery {
|
|
return query.NewConjunctionQuery(conjuncts)
|
|
}
|
|
|
|
// NewDateRangeQuery creates a new Query for ranges
|
|
// of date values.
|
|
// Date strings are parsed using the DateTimeParser configured in the
|
|
// top-level config.QueryDateTimeParser
|
|
// Either, but not both endpoints can be nil.
|
|
func NewDateRangeQuery(start, end time.Time) *query.DateRangeQuery {
|
|
return query.NewDateRangeQuery(start, end)
|
|
}
|
|
|
|
// NewDateRangeInclusiveQuery creates a new Query for ranges
|
|
// of date values.
|
|
// Date strings are parsed using the DateTimeParser configured in the
|
|
// top-level config.QueryDateTimeParser
|
|
// Either, but not both endpoints can be nil.
|
|
// startInclusive and endInclusive control inclusion of the endpoints.
|
|
func NewDateRangeInclusiveQuery(start, end time.Time, startInclusive, endInclusive *bool) *query.DateRangeQuery {
|
|
return query.NewDateRangeInclusiveQuery(start, end, startInclusive, endInclusive)
|
|
}
|
|
|
|
// NewDisjunctionQuery creates a new compound Query.
|
|
// Result documents satisfy at least one Query.
|
|
func NewDisjunctionQuery(disjuncts ...query.Query) *query.DisjunctionQuery {
|
|
return query.NewDisjunctionQuery(disjuncts)
|
|
}
|
|
|
|
// NewDisjunctionQueryMin creates a new compound Query.
|
|
// Result documents satisfy at least min Queries.
|
|
func NewDisjunctionQueryMin(min float64, disjuncts ...query.Query) *query.DisjunctionQuery {
|
|
return query.NewDisjunctionQueryMin(disjuncts, min)
|
|
}
|
|
|
|
// NewDocIDQuery creates a new Query object returning indexed documents among
|
|
// the specified set. Combine it with ConjunctionQuery to restrict the scope of
|
|
// other queries output.
|
|
func NewDocIDQuery(ids []string) *query.DocIDQuery {
|
|
return query.NewDocIDQuery(ids)
|
|
}
|
|
|
|
// NewFuzzyQuery creates a new Query which finds
|
|
// documents containing terms within a specific
|
|
// fuzziness of the specified term.
|
|
// The default fuzziness is 2.
|
|
//
|
|
// The current implementation uses Levenshtein edit
|
|
// distance as the fuzziness metric.
|
|
func NewFuzzyQuery(term string) *query.FuzzyQuery {
|
|
return query.NewFuzzyQuery(term)
|
|
}
|
|
|
|
// NewMatchAllQuery creates a Query which will
|
|
// match all documents in the index.
|
|
func NewMatchAllQuery() *query.MatchAllQuery {
|
|
return query.NewMatchAllQuery()
|
|
}
|
|
|
|
// NewMatchNoneQuery creates a Query which will not
|
|
// match any documents in the index.
|
|
func NewMatchNoneQuery() *query.MatchNoneQuery {
|
|
return query.NewMatchNoneQuery()
|
|
}
|
|
|
|
// NewMatchPhraseQuery creates a new Query object
|
|
// for matching phrases in the index.
|
|
// An Analyzer is chosen based on the field.
|
|
// Input text is analyzed using this analyzer.
|
|
// Token terms resulting from this analysis are
|
|
// used to build a search phrase. Result documents
|
|
// must match this phrase. Queried field must have been indexed with
|
|
// IncludeTermVectors set to true.
|
|
func NewMatchPhraseQuery(matchPhrase string) *query.MatchPhraseQuery {
|
|
return query.NewMatchPhraseQuery(matchPhrase)
|
|
}
|
|
|
|
// NewMatchQuery creates a Query for matching text.
|
|
// An Analyzer is chosen based on the field.
|
|
// Input text is analyzed using this analyzer.
|
|
// Token terms resulting from this analysis are
|
|
// used to perform term searches. Result documents
|
|
// must satisfy at least one of these term searches.
|
|
func NewMatchQuery(match string) *query.MatchQuery {
|
|
return query.NewMatchQuery(match)
|
|
}
|
|
|
|
// NewMatchQueryOperator creates a Query for matching text.
|
|
// An Analyzer is chosen based on the field.
|
|
// Input text is analyzed using this analyzer.
|
|
// Token terms resulting from this analysis are
|
|
// used to perform term searches. Result documents
|
|
// must satisfy term searches according to given operator.
|
|
func NewMatchQueryOperator(match string, operator query.MatchQueryOperator) *query.MatchQuery {
|
|
return query.NewMatchQueryOperator(match, operator)
|
|
}
|
|
|
|
// NewNumericRangeQuery creates a new Query for ranges
|
|
// of numeric values.
|
|
// Either, but not both endpoints can be nil.
|
|
// The minimum value is inclusive.
|
|
// The maximum value is exclusive.
|
|
func NewNumericRangeQuery(min, max *float64) *query.NumericRangeQuery {
|
|
return query.NewNumericRangeQuery(min, max)
|
|
}
|
|
|
|
// NewNumericRangeInclusiveQuery creates a new Query for ranges
|
|
// of numeric values.
|
|
// Either, but not both endpoints can be nil.
|
|
// Control endpoint inclusion with inclusiveMin, inclusiveMax.
|
|
func NewNumericRangeInclusiveQuery(min, max *float64, minInclusive, maxInclusive *bool) *query.NumericRangeQuery {
|
|
return query.NewNumericRangeInclusiveQuery(min, max, minInclusive, maxInclusive)
|
|
}
|
|
|
|
// NewPhraseQuery creates a new Query for finding
|
|
// exact term phrases in the index.
|
|
// The provided terms must exist in the correct
|
|
// order, at the correct index offsets, in the
|
|
// specified field. Queried field must have been indexed with
|
|
// IncludeTermVectors set to true.
|
|
func NewPhraseQuery(terms []string, field string) *query.PhraseQuery {
|
|
return query.NewPhraseQuery(terms, field)
|
|
}
|
|
|
|
// NewPrefixQuery creates a new Query which finds
|
|
// documents containing terms that start with the
|
|
// specified prefix.
|
|
func NewPrefixQuery(prefix string) *query.PrefixQuery {
|
|
return query.NewPrefixQuery(prefix)
|
|
}
|
|
|
|
// NewRegexpQuery creates a new Query which finds
|
|
// documents containing terms that match the
|
|
// specified regular expression.
|
|
func NewRegexpQuery(regexp string) *query.RegexpQuery {
|
|
return query.NewRegexpQuery(regexp)
|
|
}
|
|
|
|
// NewQueryStringQuery creates a new Query used for
|
|
// finding documents that satisfy a query string. The
|
|
// query string is a small query language for humans.
|
|
func NewQueryStringQuery(q string) *query.QueryStringQuery {
|
|
return query.NewQueryStringQuery(q)
|
|
}
|
|
|
|
// NewTermQuery creates a new Query for finding an
|
|
// exact term match in the index.
|
|
func NewTermQuery(term string) *query.TermQuery {
|
|
return query.NewTermQuery(term)
|
|
}
|
|
|
|
// NewWildcardQuery creates a new Query which finds
|
|
// documents containing terms that match the
|
|
// specified wildcard. In the wildcard pattern '*'
|
|
// will match any sequence of 0 or more characters,
|
|
// and '?' will match any single character.
|
|
func NewWildcardQuery(wildcard string) *query.WildcardQuery {
|
|
return query.NewWildcardQuery(wildcard)
|
|
}
|